Hi, everyone. I made a very simplistic scene manager for you all to use, its somewhat compressed as well. here's an example:
Basically, this scene manager allows you to create init, update and draw for each scene (as well as anything else you want to cook up, of course).
HOW TO USE
to make a new scene, use the command 'nscn' inside of your init function. for example: nscn({init=function() end, update=function() end, draw=function() end})
to grab the current scene thats running so you can modify it or its variables, you can simply create a local variable called 'self'.
local this=scn['self']()


I am interested in the future making a game (not even sure if it would be in Unity, Game Maker, Godot, or something else) and having some dusty arcade cabinet that you find - and it has a Pico-8 arcade game on it!
I am wondering how feasible it is for the Pico-8 game to be embedded and how well the game canvas could be moved around and positioned, incorporated into the game, to look like it's in a cabinet, etc., or if it's even possible at all.
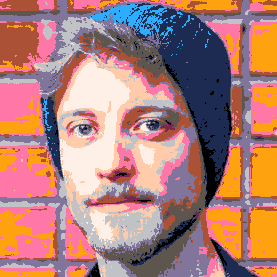

its gonna be a race
update:I added more sattelites and a cow (very productive I know).
Still thinking about the gameplay, I think I am going to make them be able to bump into eachother and collide with astroids and maybe sattelites aswell. and when they eventually get to the moon. maybe do a moonlander mini-game or something.
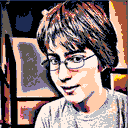


Hi all,
I'm writing a short graphical text adventure for the PICO-8, and a few weeks ago I determined that I needed a vector graphics editor capable of outputting PICO-8 code. I've created a web app called GMagic that lets you make vector drawings on a canvas, then export Lua functions for drawing them. I also provide the functions for drawing polygons and polylines:
ptstr() (needed for the functions):
Polygon:

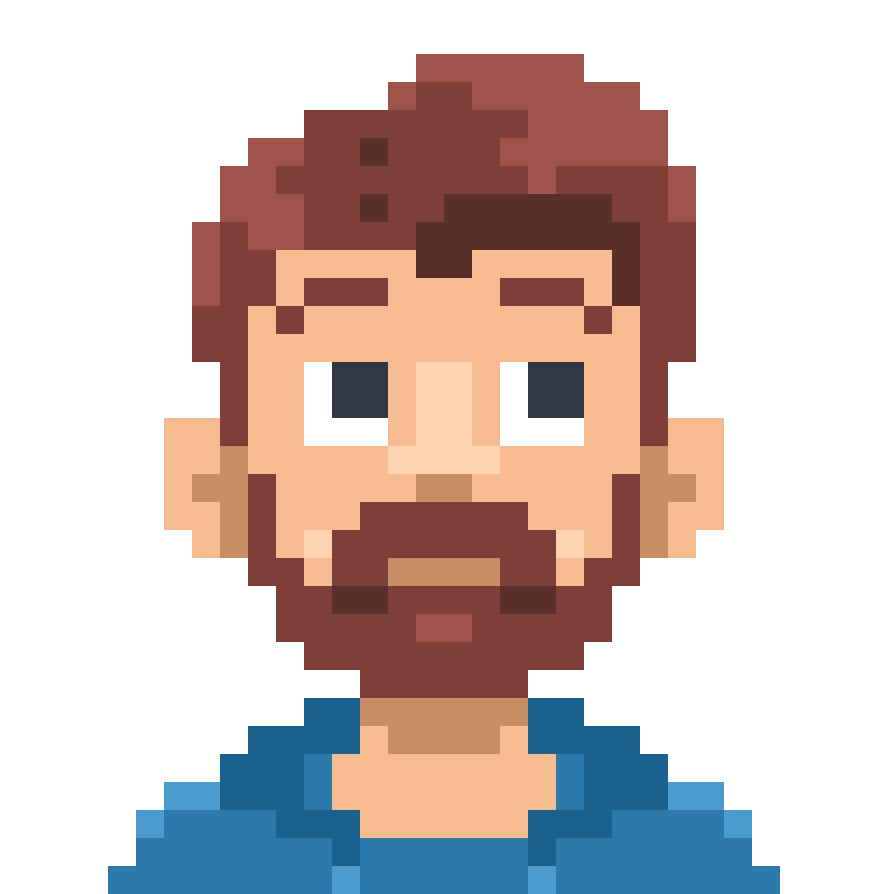
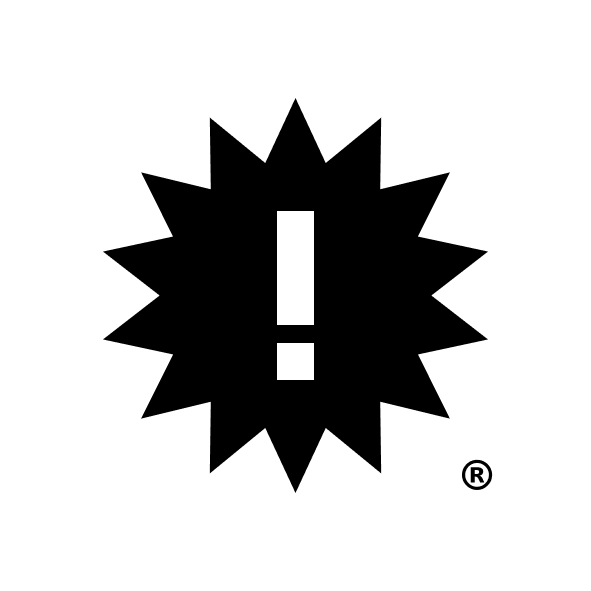

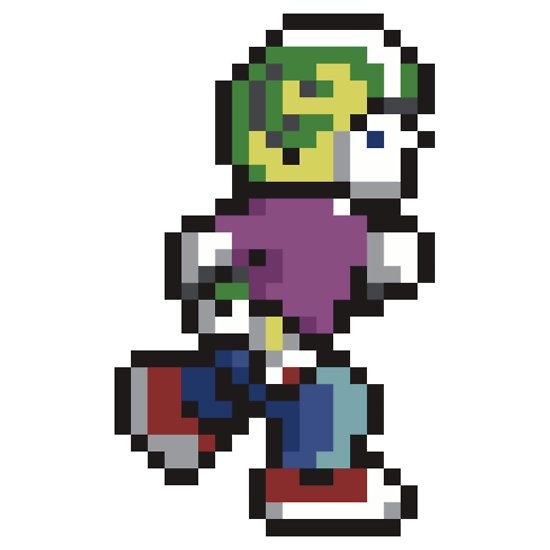
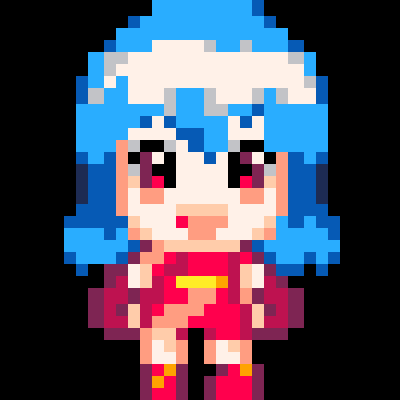
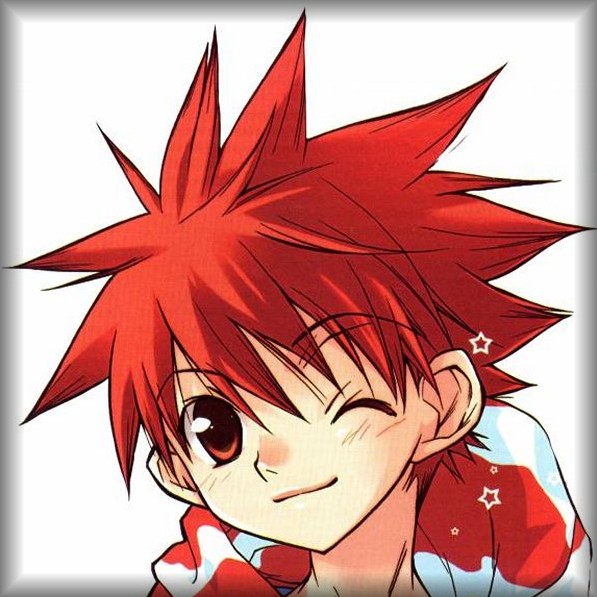


Modified previous work to add a centroid bar to spiral galaxies.
https://en.wikipedia.org/wiki/Barred_spiral_galaxy
Press: "x" to regenerate.
Instructions
- Use arrow keys to move.
- Press X to switch heroes.
- Bump enemies to attack.
- Stand on two colored squares to ascend, creating a totem from the third square.
- Stand on a green or blue totem to give the other hero an ability:
- Shoot (green) hits enemies at range.
- Jump (blue) lets you jump onto enemies and over walls.
- Step on a red totem to gain health.
- Step on an orange totem gain gold.
- Red and orange totems are refilled when an enemy steps on them.
- Ascend to the surface to win.
Inspired by Imbroglio
Thanks to @ramonaisonline for music and sounds 🙏🏻
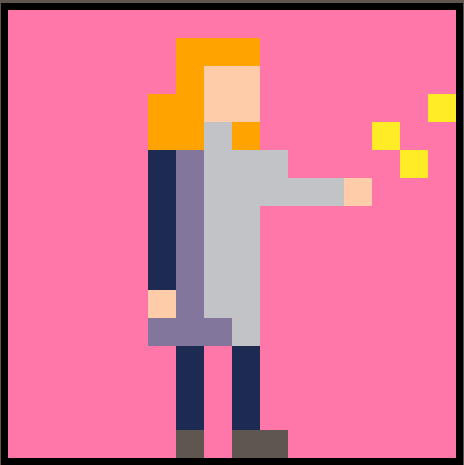

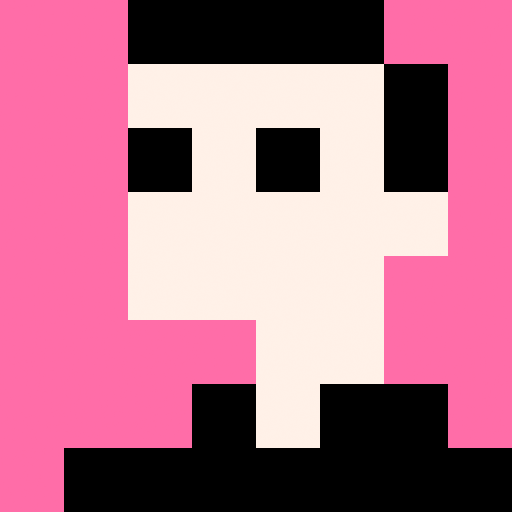

Today I am starting a new tutorial series on how to make a Roguelike in Pico-8. New episodes will be released throughout February. Hopefully, we will be done before the 7-Day Roguelike Challenge 2019 begins. The series is designed to give you the skills and tools to participate in that challenge.
If you have any questions of feedback you can post them in this thread.
Here are the videos:
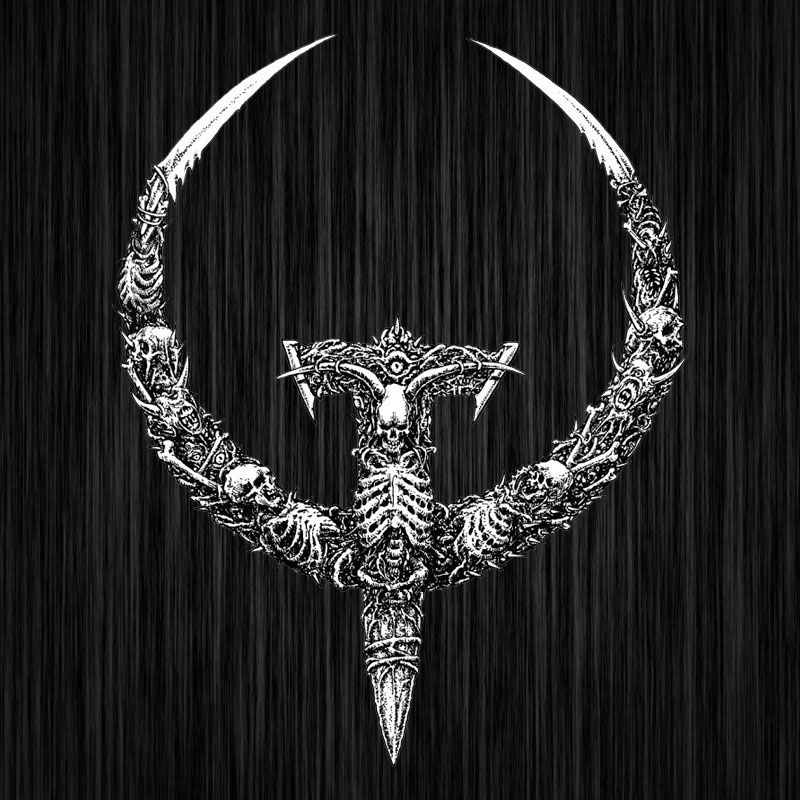

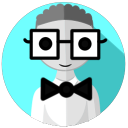

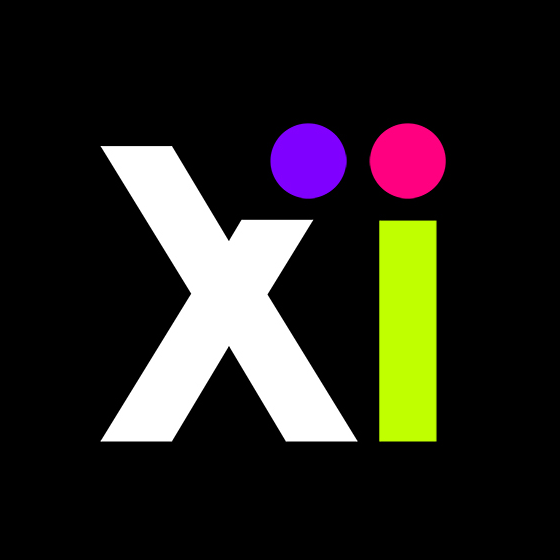
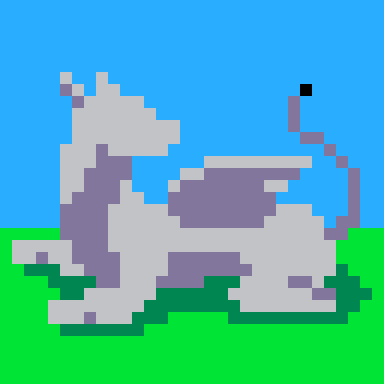
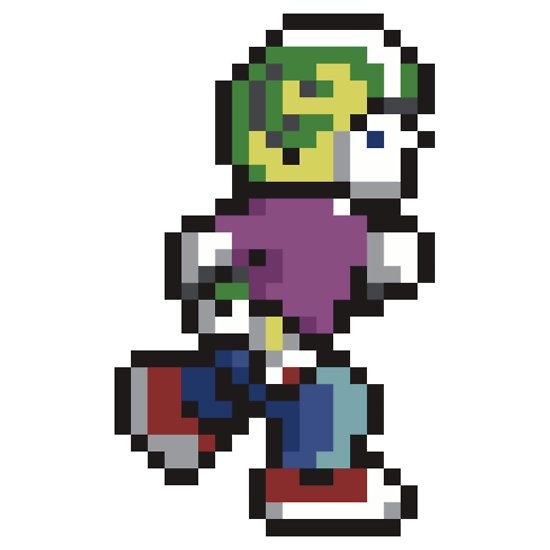
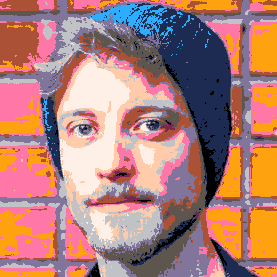

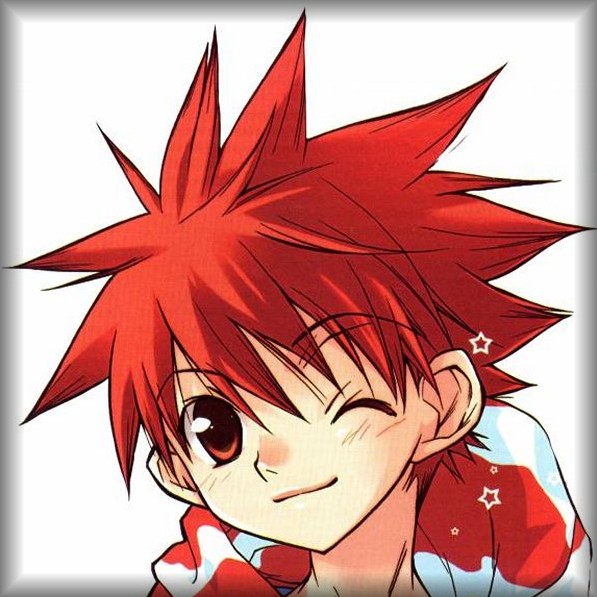
A difficult platforming game with a gardening/Splatoon-like twist. Made by Charlie Tran
This is one of my first games, and my longest to date. Please let me know what you think, thanks!
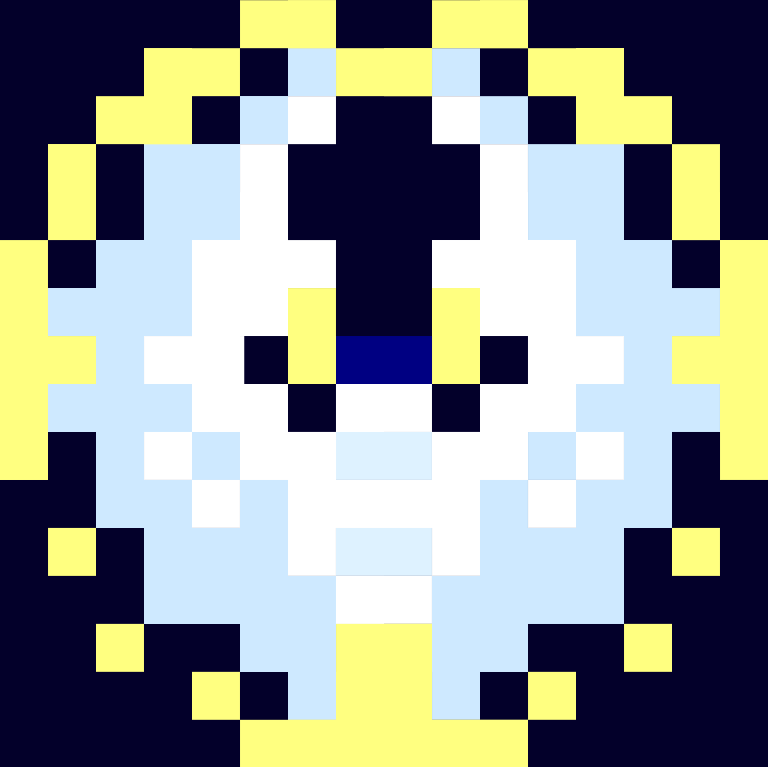

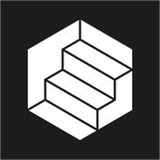

A demake of my game Patrick's Cyberpunk Challenge for the TweetTweetJam.
The object of the game is to move Patrick the Leprechaun around the board and remove all 28 squares. Squares with special symbols will remove extra squares:
Move Patrick to a square using the arrow keys. Note that you can (and sometimes must) move diagonally by pressing two arrow keys simultaneously.
Puzzles might be impossible to solve, and the game doesn't recognize if you win or lose. If the screen is empty except for Patrick, you win! If you have no legal moves, you lose and must restart the game manually.
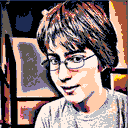
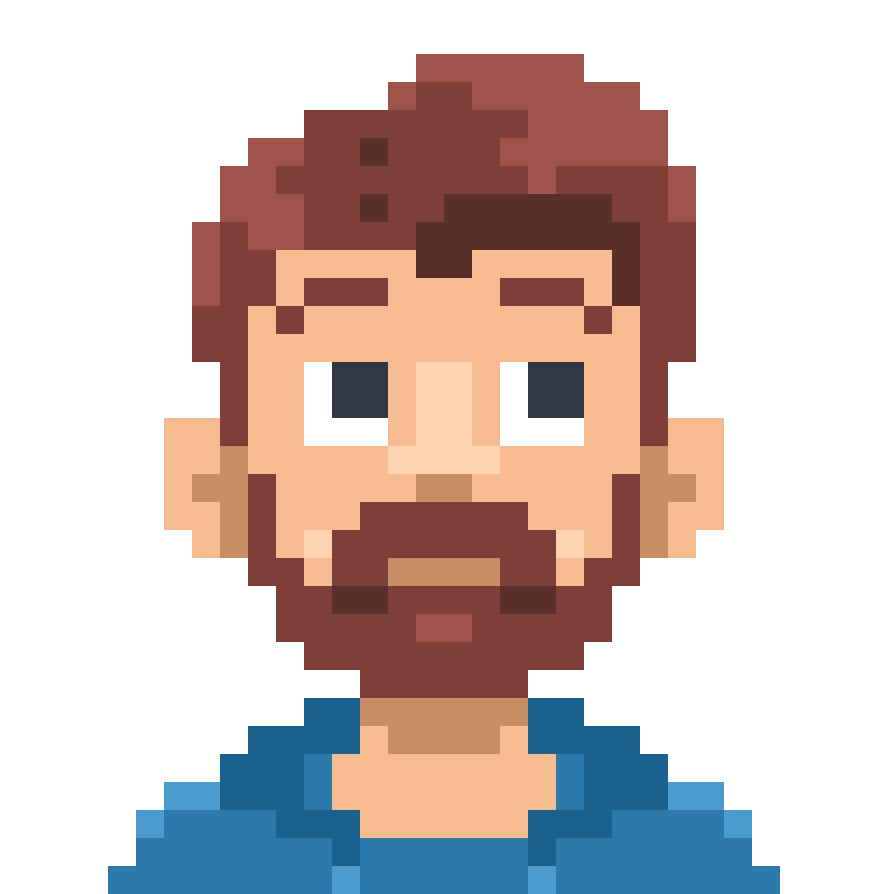
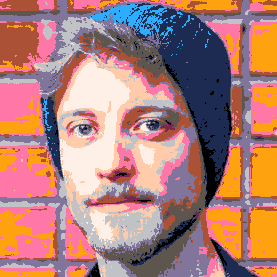
Here's a strange bug I found. If you write something like this in the code editor:
rectfill(0,0,5,5) |
and you search for 0 with CTRL+F, it will highlight the first 0. If you then press CTRL+G, however, it will fail to find the second one. The same goes for the 5.
From my testing, this seems to only happen with every other occurence. If you search for 0 here:
rectfill(0,0,0,0) |
then CTRL+G will only highlight the first and the third argument.
I've only had it happen with function arguments, and only with single-character arguments; single-letter variable names also have this problem. Putting a space after the commas also makes it behave properly.
It's a bit of a problem when writing tweetcarts where you have little whitespace, short variable names, and want to find variables or number literals to replace while optimizing :)
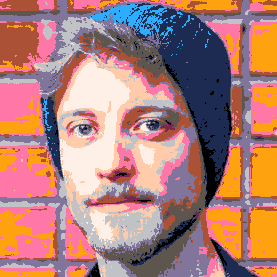